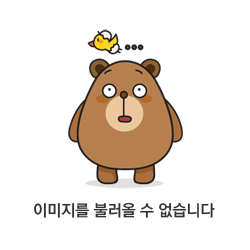
회피기 함수이름은 Dodge 를 사용해주었고
점프 중, 회피기가 나가지않도록 제약조건 != 을 걸어주었습니다.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Player : MonoBehaviour
{
public float speed;
float hAxis;
float vAxis;
bool wDown;
bool jDown;
public bool isJump;
bool isDodge;
Vector3 moveVec;
Vector3 dodgeVec;
Rigidbody rigid;
Animator anim;
void Awake() {
rigid = GetComponent<Rigidbody>();
anim = GetComponentInChildren<Animator>();
}
// Update is called once per frame
void Update()
{
GetInput();
Move();
Turn();
Jump();
Dodge();
}
void GetInput() {
//키 입력 인식
hAxis = Input.GetAxisRaw("Horizontal"); //edit-project settings-input manager-Axes 의 Horizontal
vAxis = Input.GetAxisRaw("Vertical");
wDown = Input.GetButton("Walk");
jDown = Input.GetButtonDown("Jump");
}
void Move() {
//대각선 이동 노멀라이즈
moveVec = new Vector3(hAxis, 0, vAxis).normalized;
if (isDodge) {
moveVec = dodgeVec;
}
//벡터이동
//삼항연산자 사용해서 걷기wDown true면 걷기 속도-false면 Run속도
transform.position += moveVec * speed * (wDown ? 0.3f : 1f) * Time.deltaTime;
anim.SetBool("isRun", moveVec != Vector3.zero);
anim.SetBool("isWalk", wDown);
}
void Turn() {
//키 입력에 따라 해당 방향으로 바라보기(회전)
transform.LookAt(transform.position + moveVec);
}
void Jump() {
if (jDown && moveVec == Vector3.zero && !isJump && !isDodge) { //무한점프가 안되도록 !isJump 제약조건 걸기
rigid.AddForce(Vector3.up * 16, ForceMode.Impulse); //up 방향으로 Addforce
anim.SetBool("isJump", true);
anim.SetTrigger("doJump");
//isJump = true;
}
}
void Dodge() {
if (jDown && moveVec != Vector3.zero && !isJump && !isDodge) { //무한점프가 안되도록 !isJump 제약조건 걸기
dodgeVec = moveVec;
speed *= 2;
anim.SetTrigger("doDodge");
isDodge = true;
Invoke("DodgeOut", 0.4f); //0.4초 후 DodgeOut 함수를 호출. 시간차 호출을 위해 Invoke 를 써주었습니다.
}
}
void DodgeOut() {
speed *= 0.5f;
isDodge = false;
}
private void OnCollisionStay(Collision collision) { // 캐릭터의 collision 값을 계속 디텍팅.
if(collision.gameObject.tag == "Floor") { //collision 이 닿은 오브젝트의 tag가 Floor 이면
anim.SetBool("isJump",false);
isJump = false; //점프가 가능하도록 isJump 를 false 로 반환.
}
}
private void OnCollisionExit(Collision collision) { //캐릭터와 닿은 collision과 떨어졌을때
if (collision.gameObject.tag == "Floor") { //collision 이 닿았던 오브젝트의 tag가 Floor 이면
//anim.SetBool("isJump", false);
isJump = true; //점프가 불가능하도록 isJump 를 true 로 반환.
}
}
}
출처 - https://youtu.be/eZ8Dm809j4c?list=PLO-mt5Iu5TeYI4dbYwWP8JqZMC9iuUIW2
'C' 카테고리의 다른 글
3D 쿼터뷰 액션게임 - 드랍무기 입수 (0) | 2023.06.02 |
---|---|
3D 쿼터뷰 액션게임 - 플레이어 점프 (0) | 2023.06.02 |
3D 쿼터뷰 액션게임 - 코드 정리 (0) | 2023.05.18 |
3D 쿼터뷰 액션게임 - 플레이어 이동 애니메이션, 카메라 이동 (0) | 2023.05.18 |
3D 쿼터뷰 액션게임 - 플레이어 이동 (0) | 2023.05.18 |